Azure Logic Apps - Inline JS - Convert Security Severity Ratings
Convert Microsoft severity ratings from Low, Med, High to integers if your ITSM only supports this using inline JavaScript in Logic Apps.
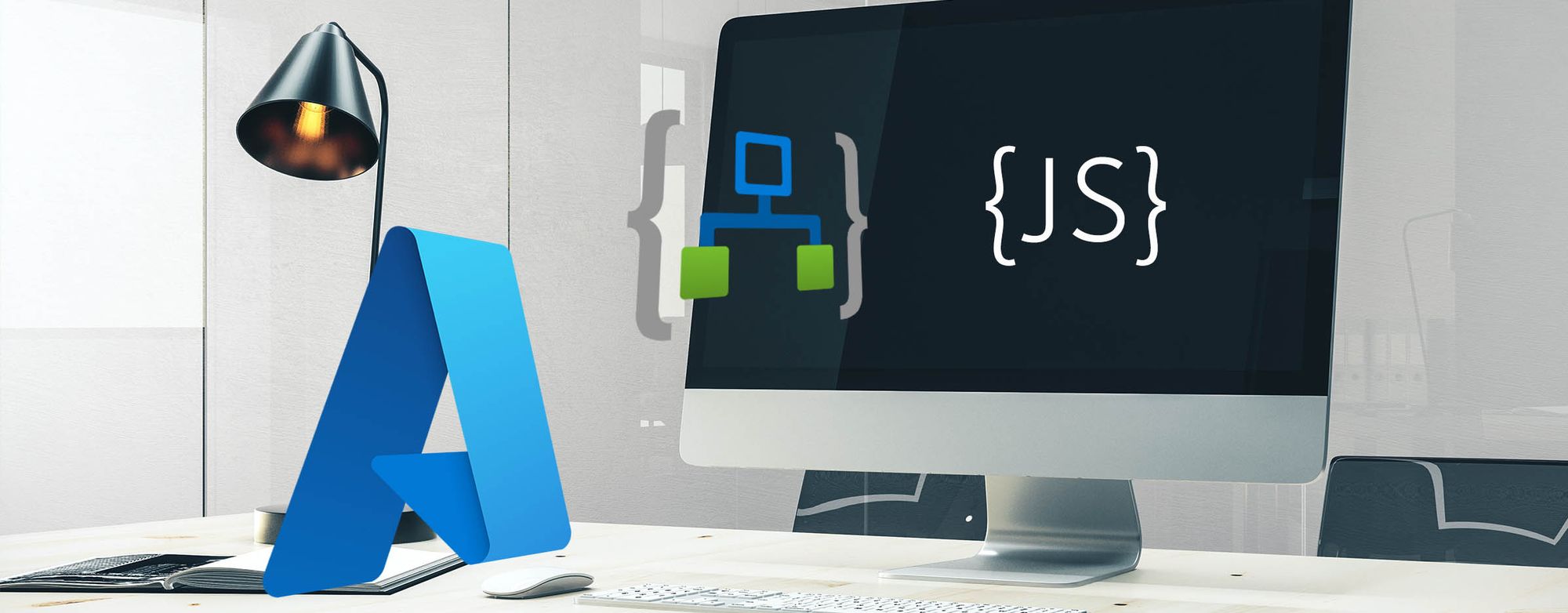
Throughout Microsoft's cloud ecosystem their severity ratings are Low, Medium and High. If you're ingesting any of these alerts whether directly from Defender for Cloud/Endpoint/Cloud Apps etc or via Sentinel then the severity might not be compatible with your ITSM such as Service Now. Unless you make another severity field dedicated to this field, but that's not ideal...
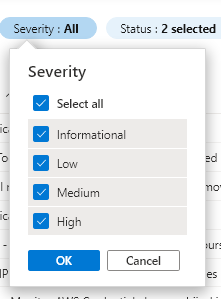
This can also work the other way round - in the project I'm currently working in I'm actually pulling down from Service Now where my severity ratings are integer and I want to convert those into Low, Medium, High to push the alert elsewhere.
All my Microsoft alerts flow through Azure logic apps and then into Service Now and I have a quick fix to replace the Low, Mediums and Highs with integers (or the other way round in this case).
Inline JavaScript
It's a very simple process and you must start by creating an integration account and adding this to the logic app you are developing. You cannot use inline JavaScript without this in place. See below:
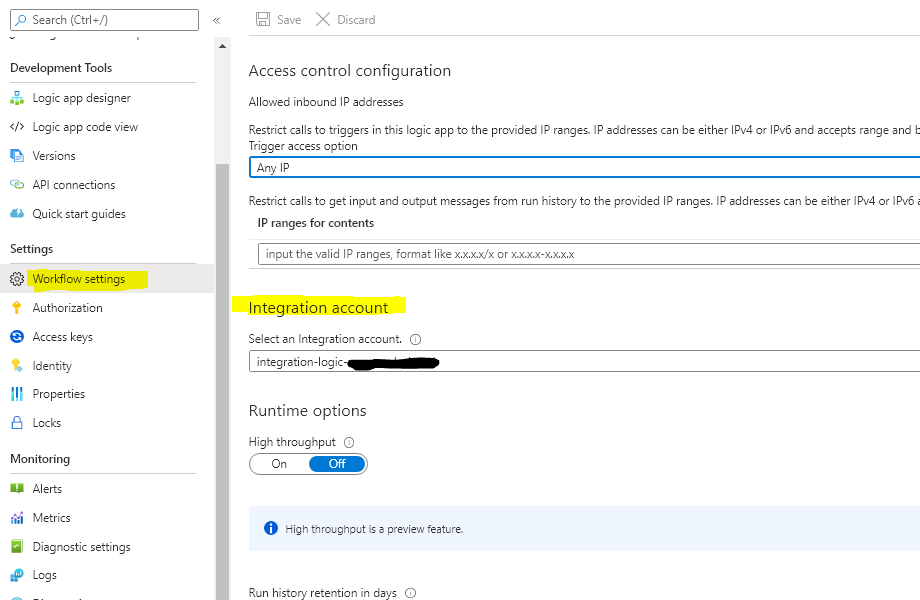
Once you've added your integration account then head to your logic app designer. Add an action and search for "javascript" or "inline code" - you should the below. Add this action to your logic.
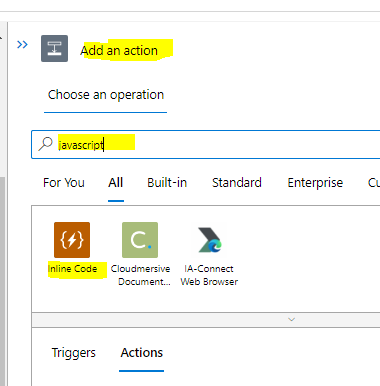
For this project my inline JS is within a For each loop as I'm dealing with more than one record each time it runs. Yours will look similar but it doesn't need to be in a loop.
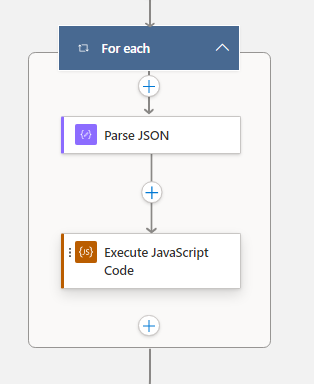
And here's the magic. In this example my previous action is a Parse JSON action but you can grab the data from any other action using "workflowContext.actions" and replacing "Parse_JSON" with the name of the action you want to take the data from. "priority" is the field name from Service Now which contains the integer. Replace this with the name of the field that contains the sev rating. This will be placed into a variable called "alertSev".
Create a new variable for the new sev rating, in this case "newAlertSev".
Then basically if statements for each rating and in this case 1 to 5.
If alertSev is equal to 1 then use JS "replace" function to swap it out for "Critical" and so on...
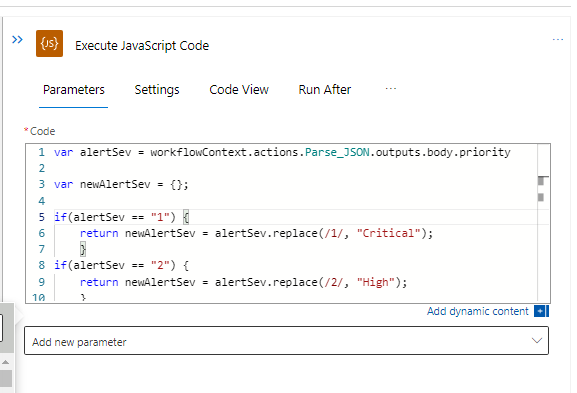
var alertSev = workflowContext.actions.Parse_JSON.outputs.body.priority
var newAlertSev = {};
if(alertSev == "1") {
return newAlertSev = alertSev.replace(/1/, "Critical");
}
if(alertSev == "2") {
return newAlertSev = alertSev.replace(/2/, "High");
}
if(alertSev == "3") {
return newAlertSev = alertSev.replace(/3/, "Medium");
}
if(alertSev == "4") {
return newAlertSev = alertSev.replace(/4/, "Low");
}
if(alertSev == "5") {
return newAlertSev = alertSev.replace(/5/, "Planning");
}
Swapping this around, if you're ingesting anything from Sentinel into your ITSM then you'll want if alertSev is equal to High then swap it out for integer "2".
var alertSev = workflowContext.actions.Parse_JSON.outputs.body.priority
var newAlertSev = {};
if(alertSev == "Critical") {
return newAlertSev = alertSev.replace(/Critical/, "1");
}
if(alertSev == "High") {
return newAlertSev = alertSev.replace(/High/, "2");
}
if(alertSev == "Medium") {
return newAlertSev = alertSev.replace(/Medium/, "3");
}
if(alertSev == "Low") {
return newAlertSev = alertSev.replace(/Low/, "4");
}
if(alertSev == "Planning") {
return newAlertSev = alertSev.replace(/Planning/, "5");
}
Test your logic
Run your logic and check the output - below we can see that this has worked successfully. I had an input of "3" for this record which was converted on the fly to "Medium". This is more human readable and I now use push this alert data elsewhere.
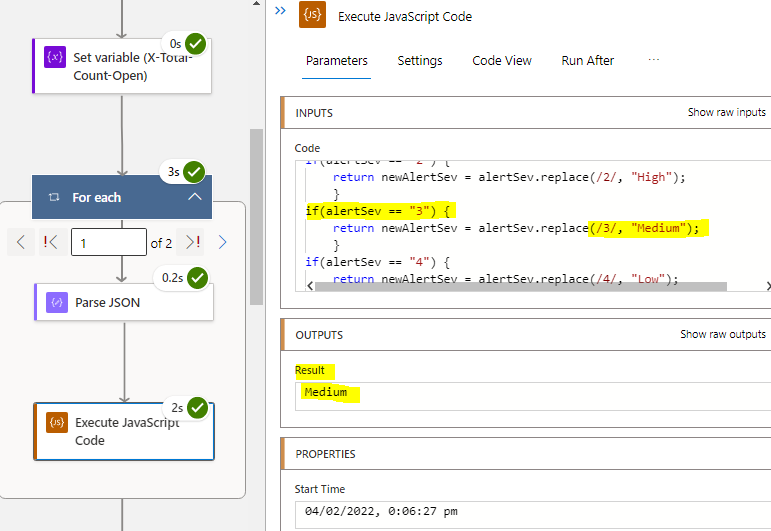
I hope this helps you. If you have any other methods for doing this I'd like to hear from you!